Generating true random strings using classical computers is not as easy as you may think. Unlike deterministic processes that follow specific algorithms and patterns, achieving true randomness poses a challenge in the realm of classical computing. Classical computers operate based on predetermined instructions and logical operations, which inherently lack the inherent unpredictability required for true randomness.
In contrast, true randomness involves an element of unpredictability that goes beyond the deterministic nature of classical computing. Attempts to generate random strings on classical computers often involve algorithms that simulate randomness, but these are ultimately constrained by the deterministic nature of the underlying hardware and software.
To achieve a higher degree of randomness, specialized hardware or external entropy sources may be employed. However, even these methods may have limitations and cannot guarantee absolute unpredictability. This is in stark contrast to quantum computers, which leverage the principles of quantum mechanics to generate truly random outcomes.
This is a small sample of code I created which uses IBM’s Quantum Computer Hardware to generate a true 4096 bit random string 🙂
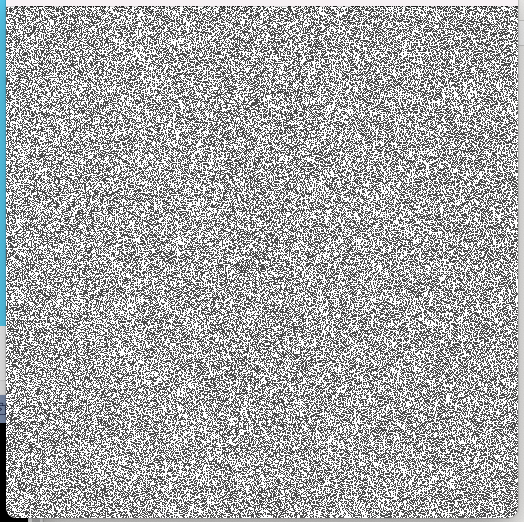
```
from qiskit import IBMQ
from qiskit_rng import Generator
provider = IBMQ.load_account()
generator = Generator(backend=provider.backends.ibmq_lima)
output = generator.sample(num_raw_bits=4096).block_until_ready()
print("Mermin correlator value is {}".format(output.mermin_correlator))
print("Raw bits are {}".format(output.raw_bits[:4096]))
int[] data;
int width = 512;
int height = 512;
void setup() {
size(512, 512);
// Load text file as a String
String[] stuff = loadStrings("123.csv");
// Convert string into an array of integers using ',' as a delimiter
data = int(split(stuff[0], ','));
//printArray(data);
}
void draw() {
background(255);
//stroke(0);
// Draw points
int k = 0;
for (int i = 0; i < width; i ++ ) {
for (int j = 0; j < height; j ++ ) {
if (data[k] == 1) {
stroke(1);
point(i, j);
}
k++;
}
}
}
```